Declaring variables in the source code is ideal when we would like to use them inside that specific source file. Let’s say we have multiple files that we would like to use the same variable in, or perhaps we would like to encrypt the value of the variable. This is when Lambda environment variables come to help.
Lambda environment variables are a key-pair of strings that are stored in a function’s version-specific configuration. The latter is important if we use versioning in Lambda. For now, we will focus on the key-pair part, we will talk about Lambda versions at a later time.
Defining environment variables
We can specify environment variables under the Configuration tab, Environment variables section.
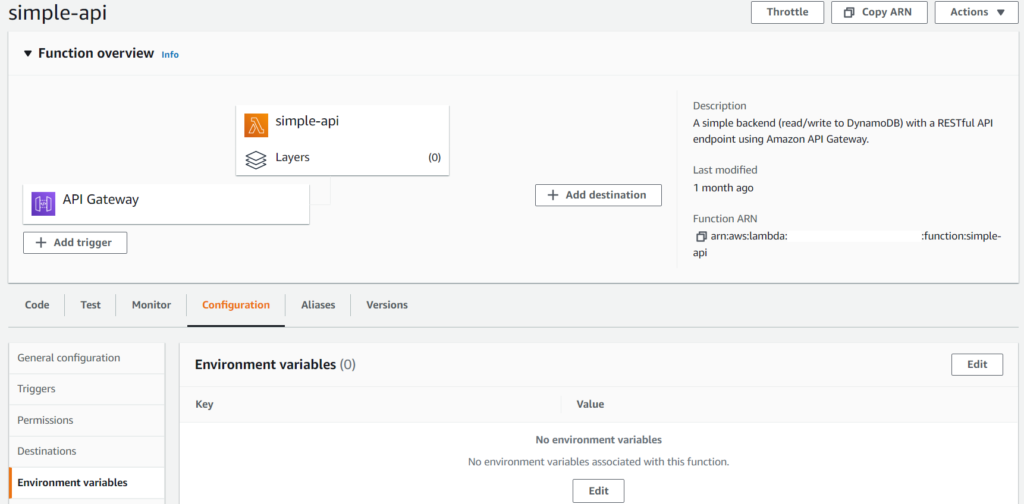
Clicking on Edit we can set the key and value of the environment variable. For this tutorial let’s create two variables: one for storing a username and another one for storing a password.
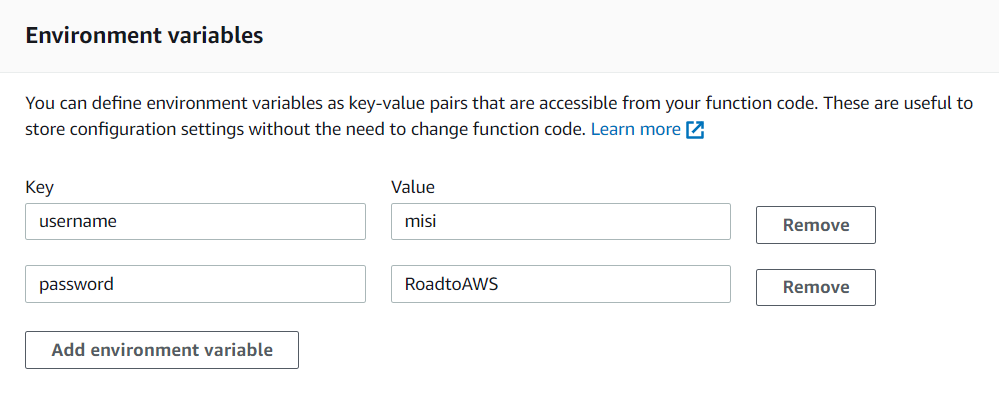
After clicking Save our environment variables are created and are available through the Lambda runtime. We can access them with the process environment, like this:
const username = process.env.username;
const password = process.env.password;
Creating a key for encryption
Our password is a piece of very sensitive information and we would like to modify our code that only our Lambda code can decrypt it. Environment variables support encryption with AWS Key Management Service (KMS).
Let’s go to the KMS Console and create a new key. Under Customer managed keys we click Create key.
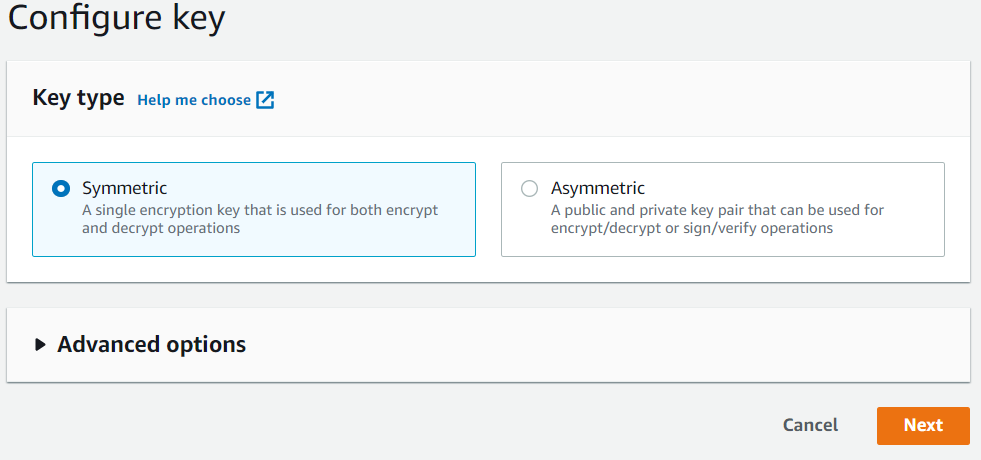
We then configure the key type to Symmetric and name our key “simple-api-key” under Alias. You can change the alias at any time. For educational purposes let’s not define key administrative permissions and key usage permissions for now.
Encrypting Lambda environment variables with KMS
Now when we go back to Lambda let’s check the Enable helpers for encryption in transit option. A new Encrypt button appears next to each variable. When clicking on Encrypt we can now select our newly created key.
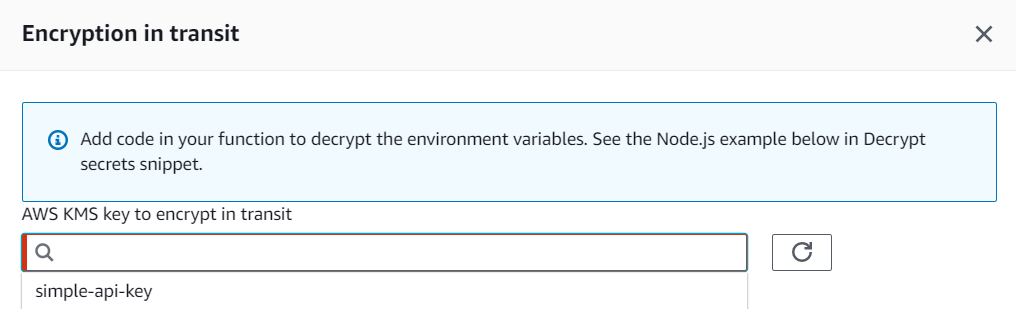
Decrypting our variable
If we look at our variable or print out it’s value from Lambda we would see something like this: AQICAHhc385PwJyf/tV5ZOhskZFcr5b6NMe/u3YFxJEWOhlnxQG776g/ozncvTV1p5KoSQucAAAAZzBlBgkqhkiG9w0BBwagWDBWAgEAMFEGCSqGSIb3DQEHATAeBglghkgBZQMEAS4wEQQMGdpuISr9cRZoNj8TAgEQgCTHd1A1f6zmXa7cCbt8Q9UJqSetCvZ6m/I8VZuLC54k/0934ZE=
In order to decrypt it we need two things:
- decrypt the variable with the KMS Decrypt operation 🔓
- grant our function permission to call the KMS Decrypt operation 🔑
First let’s modify our code to decrypt our variable. Here is a sample code:
const plainUsername = process.env.username;
const encryptedPassword = process.env.password;
let decryptedPassword;
if (!decryptedPassword) {
const kms = new AWS.KMS();
try {
const req = {
CiphertextBlob: Buffer.from(encryptedPassword, 'base64'),
EncryptionContext: {
LambdaFunctionName: process.env.AWS_LAMBDA_FUNCTION_NAME
},
};
const data = await kms.decrypt(req).promise();
decryptedPassword = data.Plaintext.toString('ascii');
} catch (err) {
console.log('Decrypt error:', err);
throw err;
}
}
When executing this code we will get an error in CloudWatch:
“errorType”:”AccessDeniedException”,”errorMessage”:”The ciphertext refers to a customer master key that does not exist, does not exist in this region, or you are not allowed to access.”
In order to decrypt the environment variable, our function needs access to the key that we used to encrypt it. Let’s go back once more to the KMS Console and modify its Key users. We now add our Lambda execution role to the key users. In our case simple-api-role.
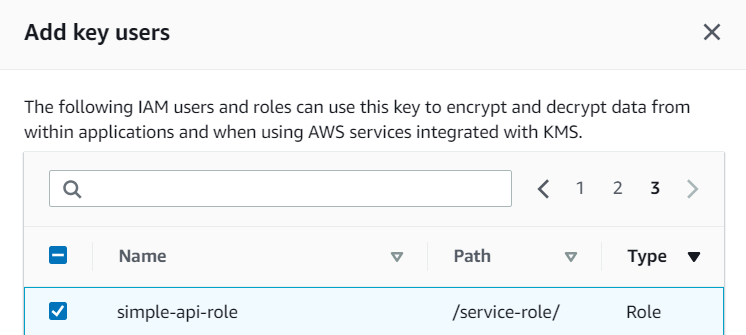
And Done! We have successfully created Lambda environment variables that we can now use in multiple source files and secured our password with KMS! 🌩️⚡